Decorators vs. Parameters: Which Comes First?
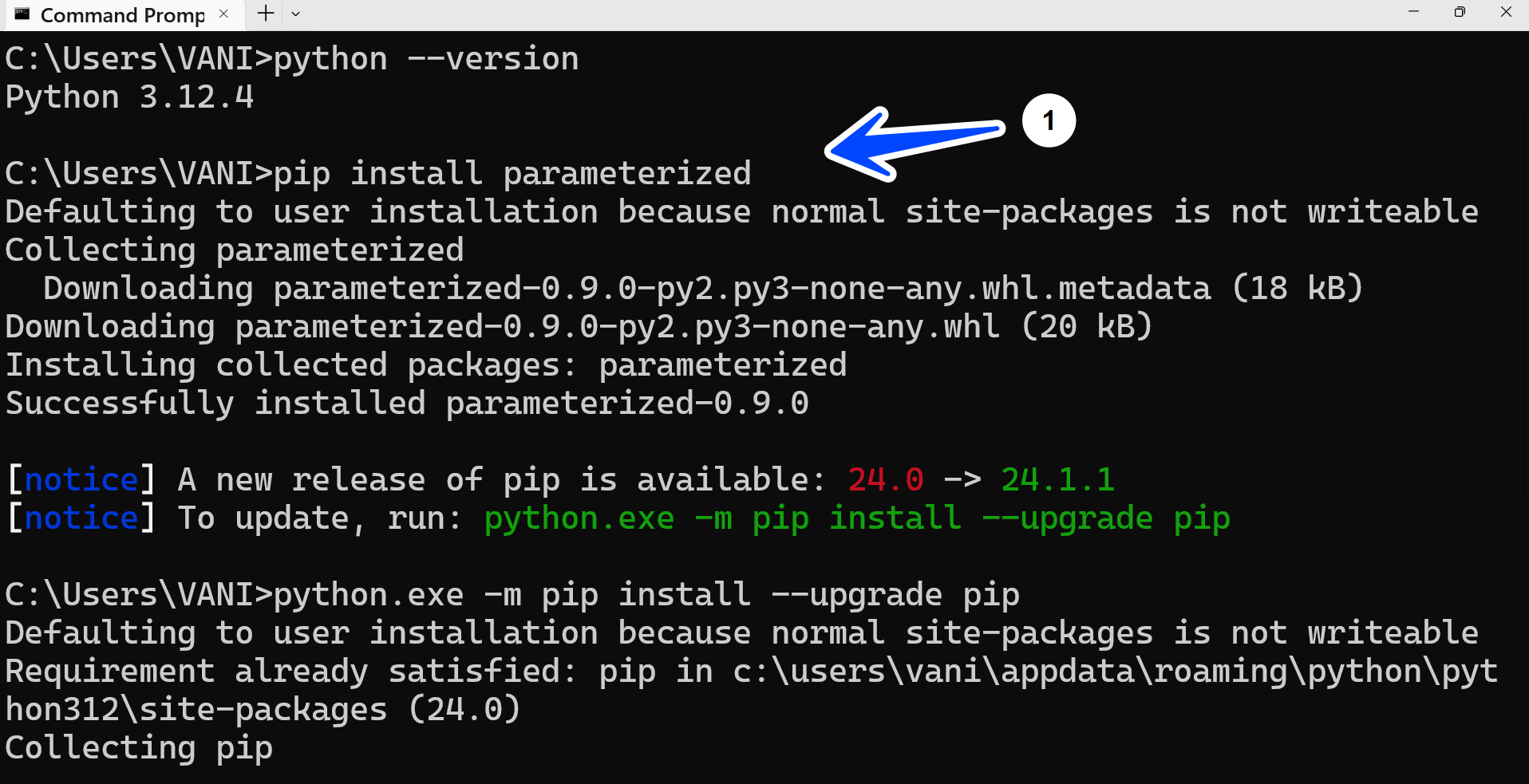
The debate between decorators and parameters in programming, specifically in languages like Python, is not just about syntax but touches upon the essence of how functions are designed, maintained, and extended over time. This exploration will dive into when to use decorators over parameters or vice versa, analyzing the advantages, limitations, and practical applications of each approach.
Defining Parameters and Decorators

Parameters are the variables listed within the parentheses when defining a function or method. They allow functions to receive data when they are called, making functions more flexible and reusable. For example:
def greet(name):
print(f"Hello, {name}!")
Here, name
is a parameter that determines who is being greeted.
Decorators, on the other hand, are a unique and powerful feature in Python. They allow you to modify or enhance functions or methods without changing their source code. A decorator is denoted by the @
symbol and wraps another function to extend or alter its behavior:
def uppercase_decorator(func):
def wrapper(*args, kwargs):
return func(*args, kwargs).upper()
return wrapper
@uppercase_decorator
def greet(name):
return f"Hello, {name}!"
In this example, the uppercase_decorator
ensures that the returned string from greet
is in all uppercase.
When to Use Parameters

- Flexibility: If your function needs data to determine its behavior or output, parameters are the way to go. They provide the function with the necessary context or data to execute its task.
- Readability: Functions with parameters make it clear at a glance what kind of data or information the function expects. This improves code readability and maintainability.
- Direct Interaction: Parameters allow for direct manipulation or interaction with the function's logic. For example, passing different thresholds to determine the severity of an alert.
When to Use Decorators

- Enhancing Functions: When you want to extend or modify the behavior of a function without changing its code or adding complexity through multiple parameters, decorators are the better choice. They can add logging, authentication, or performance tracking seamlessly.
- Reusability: Decorators can be applied to multiple functions or methods to add common functionality, promoting code reuse.
- Orthogonal Behavior: If you want to alter the function's behavior in a way that's orthogonal to its primary purpose, decorators are ideal. For instance, adding timing to a function's execution.
Comparing Decorators and Parameters in Practice

To better understand when to use decorators versus parameters, let's consider a scenario involving a hypothetical system for managing user notifications:
Parameters for Notification Content

def send_notification(user, message, priority):
print(f"Sending {message} to {user} with priority {priority}")
This function uses parameters to define the message, who to send it to, and its priority. Here, parameters give you the flexibility to customize each aspect of the notification.
Decorators for Notification Enhancements
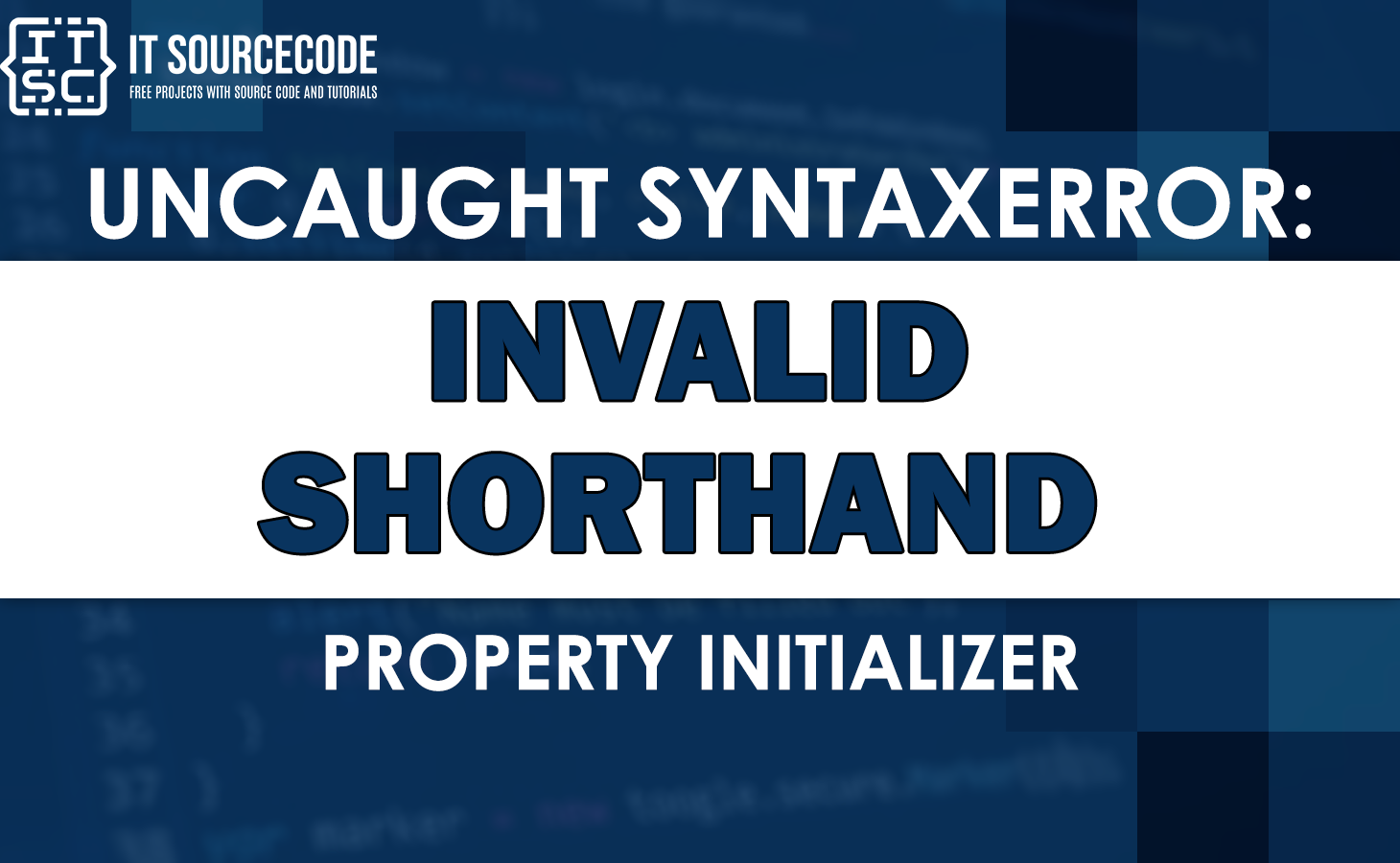
def log_notification(func):
def wrapper(*args, kwargs):
print(f"Logging notification attempt with args {args} and kwargs {kwargs}")
return func(*args, kwargs)
return wrapper
@log_notification
def send_notification(user, message, priority):
print(f"Sending {message} to {user} with priority {priority}")
Here, the log_notification
decorator adds logging functionality to the send_notification
function without altering its core logic or adding another parameter for logging purposes.
Limitations and Considerations

Parameters can:
- Lead to functions with many parameters, making them hard to manage and less readable.
- Require explicit management of data passed to functions, which might complicate the calling code.
Decorators can:
- Make the flow of execution less obvious at first glance, potentially complicating debugging.
- Add overhead due to the wrapping of functions, though usually negligible in Python.
Examples and Use Cases

Let's look at some practical scenarios:
Authentication

def requires_auth(func):
def wrapper(user, *args, kwargs):
if not user.is_authenticated:
raise ValueError("Authentication required")
return func(user, *args, kwargs)
return wrapper
@requires_auth
def get_user_data(user):
# Retrieve user data
pass
In this case, the requires_auth
decorator simplifies the authentication check without adding additional parameters to get_user_data
.
Timing Function Execution

def time_it(func):
def wrapper(*args, kwargs):
start = time.time()
result = func(*args, kwargs)
print(f"Execution time of {func.__name__}: {time.time() - start} seconds")
return result
return wrapper
@time_it
def complex_calculation():
# Some time-consuming computation
pass
Here, time_it
wraps the function to measure and print execution time without altering the function's primary logic.
Deciding Between Decorators and Parameters

The choice between using decorators or parameters often boils down to:
- Purpose of Change: If the change affects the core logic or requires specific data, parameters are more appropriate. If the change is more about orthogonal behavior or system-wide enhancements, decorators are the better fit.
- Readability and Maintainability: Consider how easy it is to understand and maintain your code when deciding. Functions with fewer parameters might be cleaner, but clear, well-commented decorators can also enhance readability.
- Reusability: Decorators shine in scenarios where the same behavior modification needs to be applied across multiple functions.
In sum, while parameters provide the basic building blocks for functions, decorators allow you to craft a more sophisticated structure. Parameters are excellent for defining the core behavior of a function, while decorators offer a way to extend and enhance functions without cluttering their interface or core logic.
🔍 Note: Keep in mind that both decorators and parameters can coexist and complement each other, enhancing the functionality of your code in different ways.
In wrapping up this discussion, it's clear that the choice between decorators and parameters isn't binary but rather a continuum. Each has its place, and their effective use can lead to cleaner, more maintainable, and expressive code. By understanding their roles, you can better decide when to decorate or when to parameterize, leading to more effective programming practices.
When should I use parameters instead of decorators?

+
Use parameters when you need to customize the behavior of a function based on specific input values or when the function’s core logic requires data to operate effectively. Parameters are straightforward and directly influence how the function processes data or makes decisions.
What are the benefits of using decorators?

+
Decorators allow for code reuse, enhance readability by separating concerns, and can modify or extend function behavior without altering the original function’s code. They are particularly useful for cross-cutting concerns like logging, authentication, or timing without affecting the primary functionality of the decorated function.
Can decorators and parameters be used together?

+
Absolutely. Decorators can enhance or modify the behavior of functions that already accept parameters. This combination allows for fine-tuning function behavior with parameters while applying reusable behavior enhancements with decorators.