5 Essential Tips to Understand Java's Decorator Pattern

In the world of software design patterns, Java's Decorator Pattern stands out as a flexible and powerful tool for enhancing object functionality dynamically. This design pattern allows developers to add new behaviors to objects without altering the existing code, making it a crucial technique for maintaining clean, modular, and extensible software systems. Here, we delve into five essential tips to help you understand and effectively use the Decorator Pattern in Java.
Understanding the Basics of Decorator Pattern

The Decorator Pattern involves a set of objects, where each object wraps another object, adding its own behavior or altering the behavior of the wrapped object. Here are the core components:
- Component: The common interface for both wrappers and concrete classes.
- Concrete Component: The original class that defines basic behavior.
- Decorator: The base decorator class that maintains a reference to a Component object.
- Concrete Decorator: Classes that extend the Decorator and add additional responsibilities.
Tip 1: Embrace Composition Over Inheritance

The Decorator Pattern exemplifies the power of composition. Rather than extending a class to inherit behavior, which can lead to a rigid and complex inheritance hierarchy, composition allows for a more flexible structure:
public interface Coffee { void prepare(); }
public class SimpleCoffee implements Coffee { @Override public void prepare() { System.out.println(“Brew coffee”); } }
public abstract class CoffeeDecorator implements Coffee { protected Coffee coffee;
public CoffeeDecorator(Coffee c) { this.coffee = c; } @Override public void prepare() { this.coffee.prepare(); }
}
public class MilkDecorator extends CoffeeDecorator { public MilkDecorator(Coffee c) { super©; }
public void prepare() { super.prepare(); System.out.println("Add milk"); }
}
public class SugarDecorator extends CoffeeDecorator { public SugarDecorator(Coffee c) { super©; }
public void prepare() { super.prepare(); System.out.println("Add sugar"); }
}
🔧 Note: This approach allows for dynamic composition of objects at runtime, enabling the creation of a virtually unlimited number of combinations.
Tip 2: Design for Single Responsibility

Each decorator should be responsible for a single aspect of functionality. This adherence to the Single Responsibility Principle (SRP) not only keeps the code cleaner but also:
- Reduces the likelihood of bugs.
- Eases maintenance.
- Makes the codebase more understandable.
Tip 3: Ensure Decorators Can Wrap Any Component

The decorator must be able to wrap any object that implements the component interface. This ensures:
- Interchangeability of components and decorators.
- A smooth path to testing, as decorators can be tested in isolation.
- Dynamic behavior adjustments without altering existing code.
✨ Note: Ensure that decorators are designed to be interchangeable with components, following the Liskov Substitution Principle.
Tip 4: Use Decorators for Optional Behavior

Decorators are particularly useful when you want to add optional or conditional behavior:
- Logging: Wrap an object to log method calls or results.
- Security: Add security checks around method calls.
- Caching: Implement caching behavior to improve performance.
Here’s an example of using decorators for logging:
public class LoggingDecorator extends CoffeeDecorator { public LoggingDecorator(Coffee c) { super©; }
public void prepare() { System.out.println("Logging start"); super.prepare(); System.out.println("Logging end"); }
}
💡 Note: Decorators can be used to add cross-cutting concerns without altering core functionalities.
Tip 5: Maintain the Interface Contract

A fundamental rule for decorators is to maintain the component’s interface. This means:
- Decorators should be invisible to clients interacting with the component.
- They must respect the same method signatures, return types, and exceptions as the component they decorate.
- This principle ensures that clients can use the decorated objects just as they would use the original components.
In wrapping up this exploration into Java's Decorator Pattern, it's clear that mastering these tips can significantly enhance your ability to design flexible, maintainable software systems. The pattern's power lies in its ability to allow developers to extend functionality dynamically while keeping the codebase clean and manageable. Whether it's for adding logging, implementing security checks, or adding any optional behavior, the Decorator Pattern offers a robust framework for solving common design problems. By embracing composition, adhering to single responsibility, ensuring decorators wrap any component, using decorators for optional behavior, and maintaining the interface contract, developers can leverage this pattern to its fullest potential, promoting code that is not only easier to extend but also easier to maintain and understand.
What is the primary benefit of using the Decorator Pattern in Java?
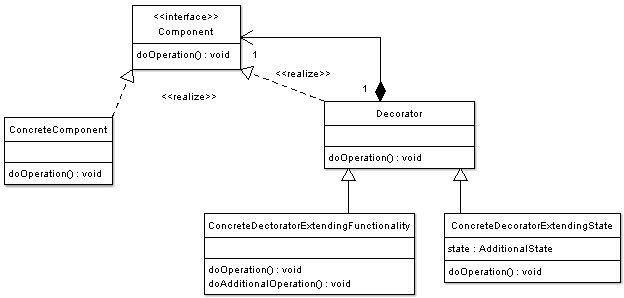
+
The primary benefit of using the Decorator Pattern is that it allows you to dynamically add behavior to an object without affecting other objects or altering the existing code base. This pattern supports the Open-Closed Principle, making software systems more extensible and maintainable.
Can decorators be stacked in Java?

+
Yes, decorators can be stacked. One decorator can wrap another, allowing for complex combinations of behaviors. This stacking creates a layered approach to adding functionality.
How does the Decorator Pattern relate to inheritance?

+
The Decorator Pattern uses composition instead of inheritance to extend functionality. This makes it more flexible than inheritance, as it avoids issues like the “diamond problem” and allows for runtime changes in behavior.