When to Use Decorator Pattern in Java: Explained Simply

Understanding when to apply design patterns in Java can greatly improve the design, readability, and maintainability of your code. Among various design patterns, the Decorator Pattern stands out for its unique ability to dynamically add new behaviors to an object without affecting other objects of the same class. This article will delve into when and how you should use the Decorator Pattern in Java, highlighting its benefits and providing practical examples.
Understanding the Decorator Pattern

The Decorator Pattern allows for the extension of an object's behavior in a flexible way by wrapping it in another object which adds its own behaviors. Here's a simple analogy: think of it like adding toppings to a pizza. Each topping you add to the pizza modifies it but does not change the nature of the base pizza.
- Component Interface: This is an interface or an abstract class defining the operations that the object can perform.
- Concrete Component: The object to which additional responsibilities can be attached.
- Decorator: An abstract class with a reference to a Component object and implements the Component interface.
- Concrete Decorators: These classes implement the added responsibilities to the component.
When to Use the Decorator Pattern

1. When You Need to Add New Functionalities to Objects Dynamically

One of the most common scenarios where the Decorator Pattern shines is when you need to add functionalities to objects at runtime. Instead of subclassing and creating a new class for each combination of additional behaviors, you can use decorators to dynamically add behaviors. For instance:
- In a GUI framework, where you might want to add scroll, border, or resizing capabilities to components without changing their basic functionality.
2. When Subclassing Would Create Too Many Classes

If you find that your class hierarchy is exploding because of every possible combination of features or behaviors, the Decorator Pattern can help. Instead of creating multiple classes, decorators allow you to:
- Build objects incrementally, only adding the behaviors you need at runtime.
- Avoid the exponential growth of subclasses by composing objects with decorators.
3. When Changes Should Be Transparent to the Object’s Clients

The Decorator Pattern ensures that clients of the component classes do not see any difference between a component wrapped in decorators and a simple component. This makes changes transparent:
- This is particularly useful in cases where the behavior of an object needs to be modified without changing the code that uses the object.
4. For Single Responsibility Principle (SRP)

Decorators help in adhering to the single responsibility principle by separating the core responsibilities of a class from additional behaviors:
- The core functionality remains within the class, while additional responsibilities can be managed externally.
5. To Allow for Cross-Cutting Concerns
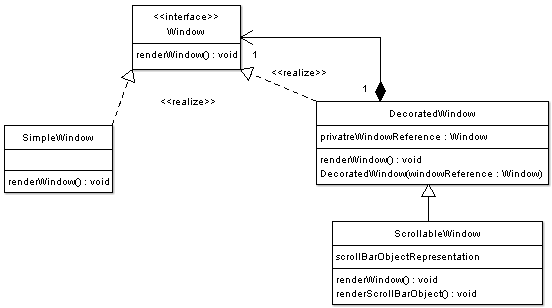
In scenarios where functionalities like logging, security, or performance monitoring need to be added:
- Decorators allow these cross-cutting concerns to be added without cluttering the original classes.
Practical Example: Enhancing User Interface Components

Let's consider a simple example where we want to enhance UI components with additional features like borders, shadows, and focus effects:
// Component Interface
interface UIComponent {
void display();
}
// Concrete Component
class Button implements UIComponent {
@Override
public void display() {
System.out.println("Displaying Button");
}
}
// Decorator Abstract Class
abstract class UIComponentDecorator implements UIComponent {
protected UIComponent component;
public UIComponentDecorator(UIComponent component) {
this.component = component;
}
public void display() {
component.display();
}
}
// Concrete Decorator for Border
class BorderDecorator extends UIComponentDecorator {
public BorderDecorator(UIComponent component) {
super(component);
}
@Override
public void display() {
super.display();
addBorder();
}
private void addBorder() {
System.out.println("Adding Border to UI Component");
}
}
// Concrete Decorator for Shadow
class ShadowDecorator extends UIComponentDecorator {
public ShadowDecorator(UIComponent component) {
super(component);
}
@Override
public void display() {
super.display();
addShadow();
}
private void addShadow() {
System.out.println("Adding Shadow to UI Component");
}
}
🔍 Note: Here, we've kept the example concise but in real-world scenarios, decorators could include more complex behaviors or even involve different types of UI components.
Now you can create an instance of a button with additional features like this:
UIComponent decoratedButton = new ShadowDecorator(new BorderDecorator(new Button()));
decoratedButton.display();
// Output:
// Displaying Button
// Adding Border to UI Component
// Adding Shadow to UI Component
This demonstrates how decorators can enhance the behavior of a `Button` component by adding borders and shadows dynamically, while keeping the `Button` class itself unchanged.
Key Benefits and Considerations

Benefits:

- Flexibility: Allows for a wide range of permutations without subclassing.
- Maintains Open-Closed Principle: Classes are open for extension but closed for modification.
- Clean Code: Reduces the complexity of the class hierarchy.
Considerations:

- The pattern can result in a large number of small objects in the system, which might complicate debugging.
- Creating the right combination of decorators might require careful consideration to avoid performance issues.
The end of this exploration into the Decorator Pattern in Java reveals its strategic importance for extending object capabilities without modifying their core structure. By adding behaviors dynamically, programmers can achieve enhanced flexibility in software design, maintainability, and adherence to the principles of object-oriented programming. With the ability to layer multiple decorators, you not only modularize your code but also facilitate the addition of responsibilities at runtime. This approach not only simplifies the software's structure but also makes it more adaptable to changing requirements, allowing for a cleaner, more intuitive codebase that can evolve seamlessly with your project's growth.
What makes the Decorator Pattern different from the Adapter Pattern?

+
The Decorator Pattern adds responsibilities to an object at runtime, whereas the Adapter Pattern helps objects with incompatible interfaces work together by wrapping an existing class with a new interface. Decorators maintain the interface, while adapters change it.
Can a class be decorated with multiple decorators?

+
Yes, you can stack decorators to add multiple behaviors. Each decorator wraps around the component or another decorator, creating a chain of decorators that enhance the base component’s capabilities.
What are the potential downsides of using the Decorator Pattern?

+
While powerful, the Decorator Pattern can lead to complexity due to the creation of numerous small objects, which might be difficult to debug. Additionally, excessive use can lead to performance overhead due to the chaining of decorators.