Why Decorator Pattern Avoids Subclassing Explained

When developing software, developers often face the challenge of extending the behavior of existing classes without altering their core functionalities. This is where design patterns like the Decorator Pattern come into play. This pattern provides a flexible alternative to subclassing for extending functionality in object-oriented programming. Let's delve into how the Decorator Pattern avoids subclassing and why it's advantageous in many programming scenarios.
Understanding Subclassing
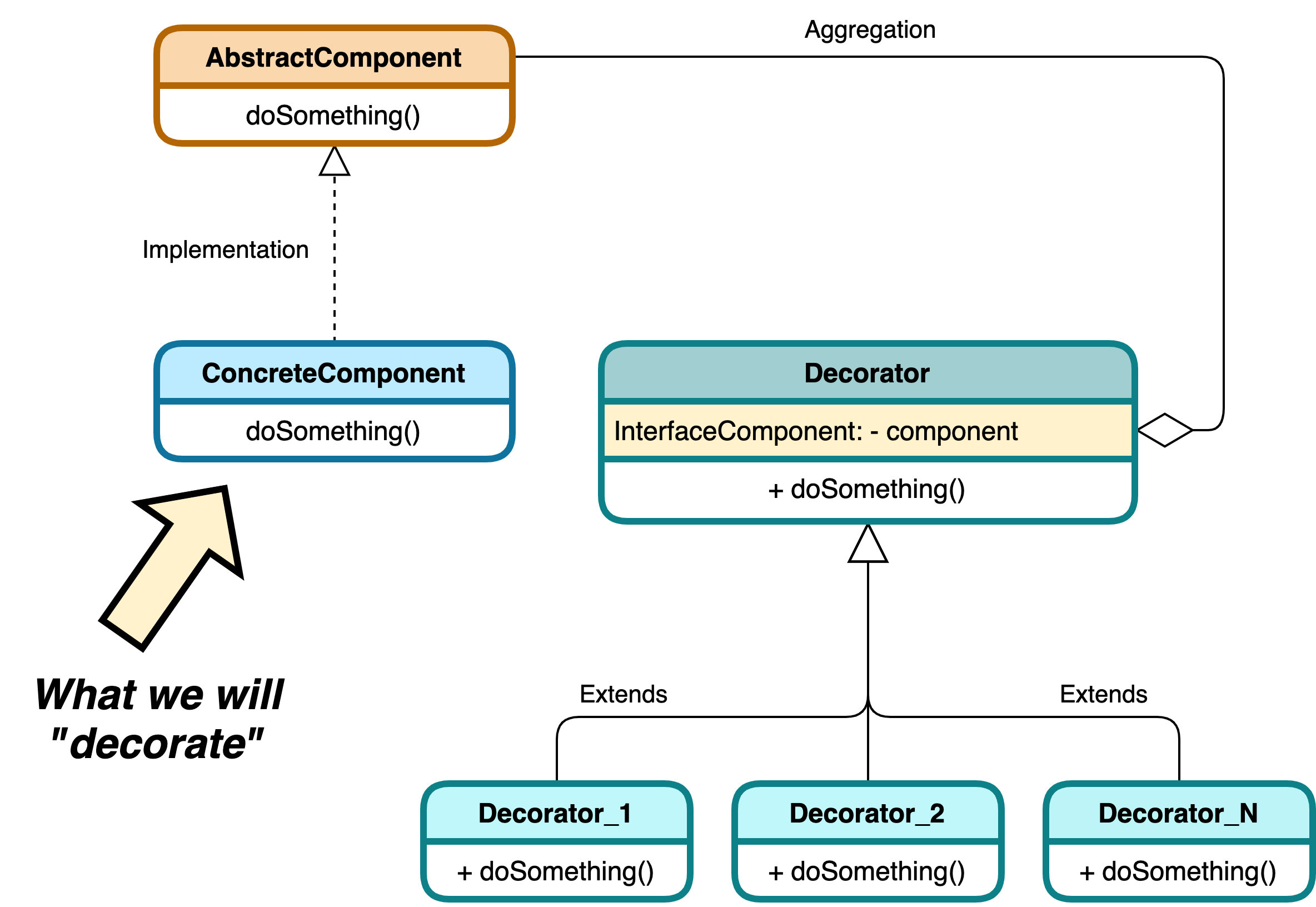
Subclassing, or inheritance, is a fundamental principle in object-oriented programming where a new class inherits properties and methods from an existing class. While it’s straightforward and effective for adding functionality to a single class or a small hierarchy:
- It can lead to a proliferation of classes: Each new variation requires its subclass, resulting in a rigid, complex structure.
- The inheritance hierarchy becomes unmanageable: As new features are added, the inheritance tree grows, making code maintenance difficult.
The Decorator Pattern addresses these issues by offering a way to add responsibilities to objects at runtime without affecting other objects from the same class.
What is the Decorator Pattern?

The Decorator Pattern involves:
- A base component interface or abstract class - This defines the contract for objects that can have responsibilities added to them dynamically.
- Concrete components - Classes implementing this interface or extending this abstract class.
- A decorator base class - Conforms to the interface or extends the abstract class and contains a reference to a component.
- Concrete decorators - Extends the decorator base class and adds new behaviors by defining new methods or enhancing existing ones.

How the Decorator Pattern Works
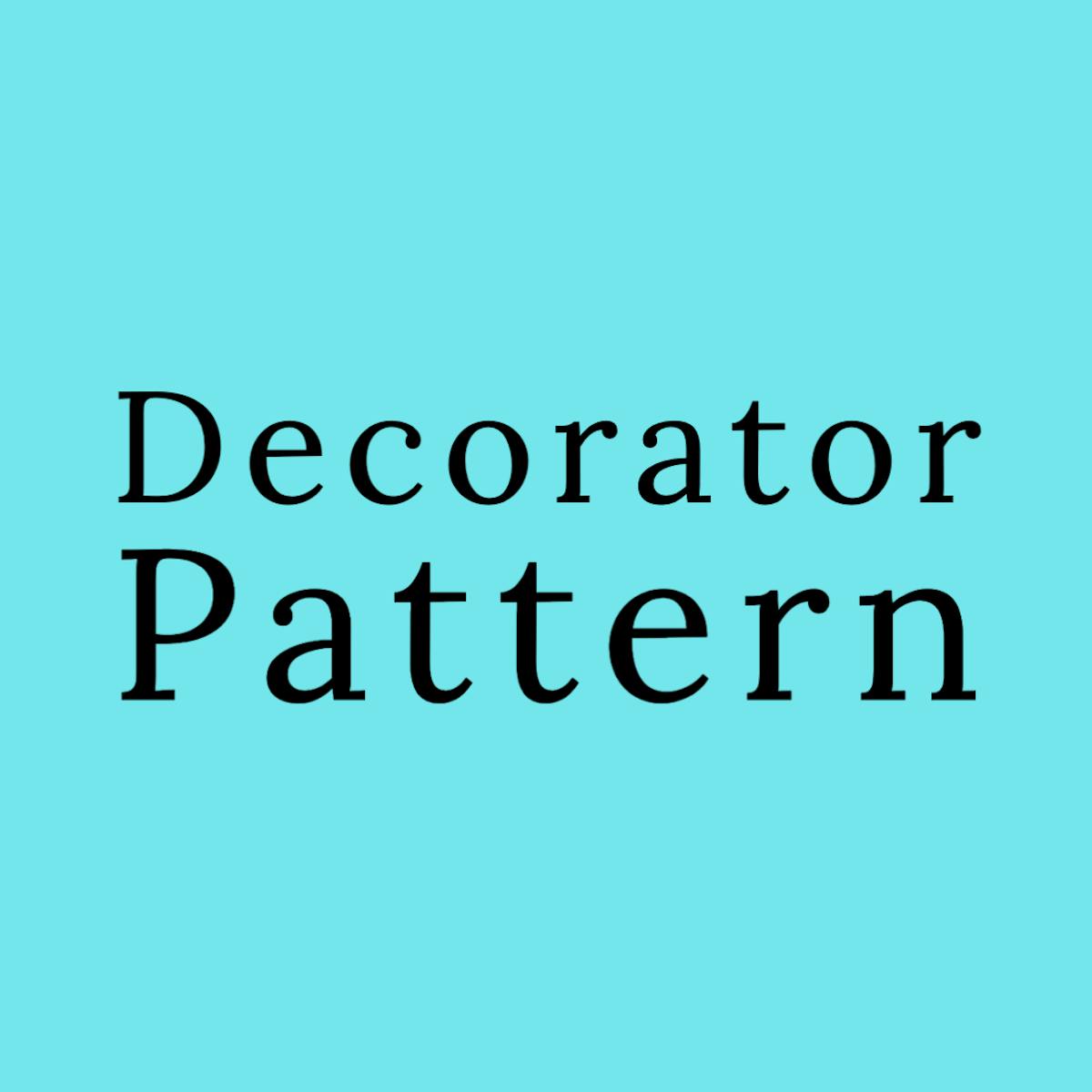
In practice, decorators:
- Wrap an original component and delegate calls to it while adding new functionalities.
- Can be stacked or nested to combine multiple behaviors.
- Do not affect the component itself, only its behavior at runtime.
Consider a simple text editor application. Without using subclassing, implementing different text formatting options (like bold, italic, underline) would require creating a new subclass for each combination. With the Decorator Pattern:
- We define a
Text
interface:
```html
- We create a concrete
PlainText
class:
```html
- Then, we design a decorator base class
TextDecorator
:
```html
- And concrete decorators like
BoldTextDecorator
andItalicTextDecorator
:
```html
public interface Text {
String getFormattedText();
}
```
public class PlainText implements Text {
private String content;
public PlainText(String content) {
this.content = content;
}
@Override
public String getFormattedText() {
return content;
}
}
```
public abstract class TextDecorator implements Text {
protected Text text;
public TextDecorator(Text text) {
this.text = text;
}
@Override
public String getFormattedText() {
return text.getFormattedText();
}
}
```
public class BoldTextDecorator extends TextDecorator {
public BoldTextDecorator(Text text) {
super(text);
}
@Override
public String getFormattedText() {
return "" + super.getFormattedText() + " ";
}
}
public class ItalicTextDecorator extends TextDecorator {
public ItalicTextDecorator(Text text) {
super(text);
}
@Override
public String getFormattedText() {
return "" + super.getFormattedText() + " ";
}
}
```
This design allows for dynamic formatting without subclassing, as we can decorate a plain text with bold, italic, or both functionalities at runtime:
```html
Text text = new PlainText("Hello, World!");
text = new BoldTextDecorator(text);
text = new ItalicTextDecorator(text);
System.out.println(text.getFormattedText());
// Output: Hello, World!
```
⚠️ Note: This example uses HTML tags for demonstration; actual formatting would depend on the text editor's implementation or UI framework.
Benefits of Using Decorators Over Subclassing

- Minimizes class proliferation - Decorators add behavior without creating new classes for each combination.
- Flexibility in modifying behaviors - Add, remove, or alter behaviors dynamically.
- Open/Closed Principle - Classes are open for extension but closed for modification.
- Orthogonal features - Behaviors like bold and italic can be used in any combination without conflicts.
When to Use the Decorator Pattern

Consider using the Decorator Pattern when:
- You need to attach additional responsibilities to an object dynamically.
- Subclassing is impractical or leads to an unwieldy class hierarchy.
- You want to alter the behavior of individual objects without affecting other objects of the same class.
- When extending behavior via subclassing would create too many classes or introduce multiple inheritance issues.
Challenges and Considerations

The Decorator Pattern is not without its challenges:
- Understanding and learning curve - The pattern can be complex for developers new to the concept.
- Additional complexity - More classes and interfaces can make the codebase seem more complex at first glance.
- Potential for debugging difficulties - With multiple decorators, it can be challenging to follow the flow of the program.
Addressing these concerns involves good documentation, sensible naming, and keeping the decorator chain as simple as possible. Additionally, careful design can ensure that the benefits of the Decorator Pattern far outweigh its downsides.
Wrapping Up

To sum up, the Decorator Pattern provides a sophisticated solution for extending the behavior of objects in a way that avoids the rigidity of subclassing. By allowing the dynamic addition of responsibilities to objects, it enhances software maintainability, reduces the complexity of class hierarchies, and supports clean and modular code design.
In software development, choosing the right design pattern can significantly impact the project’s scalability, flexibility, and ease of maintenance. The Decorator Pattern, in particular, shines when applied to problems where adding new functionality needs to be done flexibly and without the need to alter the original classes or multiply subclasses excessively.
Why would I choose the Decorator Pattern over subclassing?
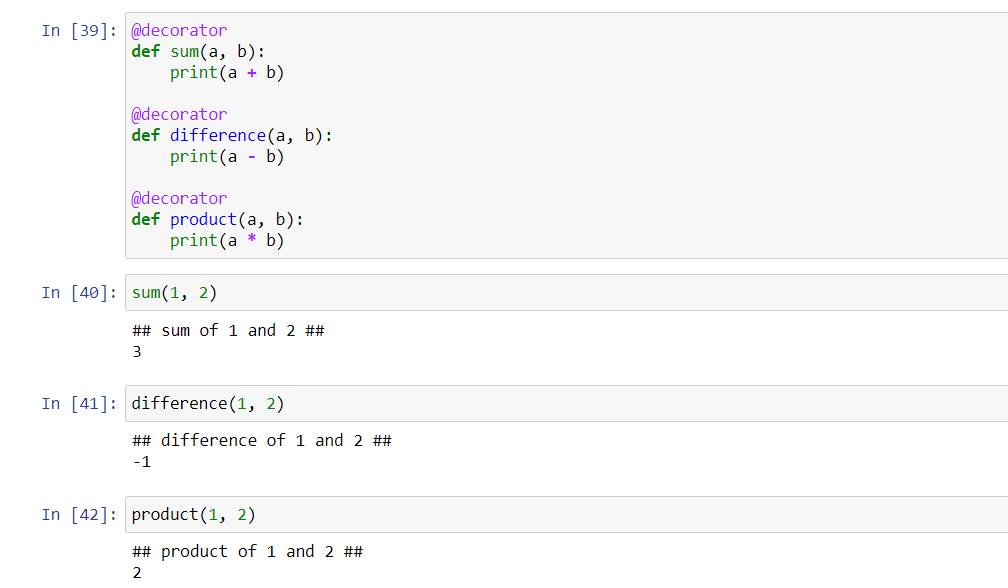
+
You’d choose the Decorator Pattern when you need to add responsibilities to objects at runtime or when subclassing would lead to an unwieldy proliferation of classes. It’s especially useful for orthogonal features where you want combinations of behaviors without creating separate subclasses for each combination.
Can decorators be removed at runtime?

+
Yes, since decorators are separate objects, you can remove them at runtime or reassign different decorators, which is something you can’t easily do with static subclassing.
Is there any performance impact when using the Decorator Pattern?

+
Yes, there can be a slight performance impact due to the overhead of additional method calls through the chain of decorators. However, this overhead is typically negligible unless the code is extremely performance-sensitive.
How do decorators work with object-oriented principles?

+
Decorators adhere to the Open/Closed Principle (open for extension, closed for modification) and support the Single Responsibility Principle by allowing classes to be designed to focus on one thing. They also help in following the Liskov Substitution Principle by maintaining type consistency.
Can I use the Decorator Pattern in other programming paradigms besides OOP?

+
While the Decorator Pattern is deeply rooted in object-oriented programming, concepts similar to decorators exist in functional programming languages like decorators in Python or higher-order functions. However, the pattern’s true intent, which involves runtime composition of behavior, is most aligned with OOP.