Decorator Classes: Inheritance Explained Simply
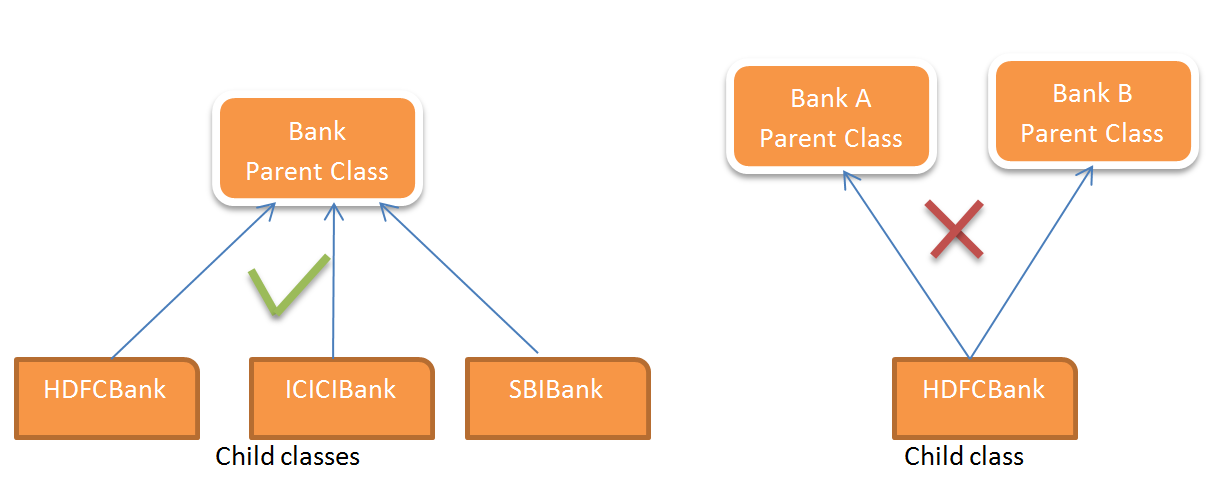
What are Decorator Classes?

Decorator classes are design patterns that allow for the dynamic addition of behavior to existing objects without altering their structure. This pattern falls under the structural category in design patterns, making it easier for developers to add functionalities to an individual object, either statically or dynamically.
Imagine you have a coffee shop where you make standard espresso, and customers can add toppings like steamed milk, foam, or syrup. Rather than making a new class for each combination, decorators help you add these toppings as you go. Each decorator corresponds to a topping or modification, wrapping around the core object.
Why Use Decorator Classes?

The use of decorators offers multiple benefits:
- Flexibility: Add features to specific objects, not all instances of a class.
- Maintainability: Enhances the ability to manage complexity in large applications.
- Single Responsibility Principle: Each decorator class is dedicated to one behavior or addition.
- Extensibility: You can extend an object's behavior without needing to subclass it or alter its core structure.
How Decorators Work

The concept behind decorators is to take an object, wrap it in a new class, and then modify or enhance its behavior. Here’s how you can implement this:
- Component: The core interface or base class which defines the operations to be performed on objects.
- ConcreteComponent: The actual object we want to decorate.
- Decorator: The base class that implements the same interface as the Component and contains a reference to a Component instance.
- ConcreteDecorator: A decorator class that extends the Decorator class and adds additional responsibilities to the Component.
Here's an example of how this might work in a coffee shop:
Class | Description |
---|---|
Beverage | Defines the interface for making a drink. |
Espresso | A concrete class for making an espresso. |
BeverageDecorator | A decorator that wraps around a Beverage. |
Milk | A ConcreteDecorator that adds milk to a beverage. |
Syrup | Another ConcreteDecorator for adding syrup to a beverage. |

💡 Note: This structure allows for stacking multiple decorators on a single object, providing a flexible and dynamic approach to object composition.
Decorator Classes and Inheritance

While decorators are often associated with composition over inheritance, they don't eliminate inheritance entirely. Here's how inheritance fits into the decorator pattern:
Inheritance for Interface Compliance

Both the decorator and component classes inherit from a common interface or base class to ensure they can be used interchangeably. This allows the client code to work with any object that implements this interface, whether it's a simple beverage or a highly decorated one.
Inheritance for Base Decorator

When creating the decorator base class, it inherits from the component's interface or class. This way, the decorator can behave exactly like the component but with additional responsibilities:
// Java Example
public abstract class BeverageDecorator extends Beverage {
protected Beverage beverage;
public BeverageDecorator(Beverage beverage) {
this.beverage = beverage;
}
}
In this case, BeverageDecorator
inherits from Beverage
to ensure it matches the interface or base class, allowing it to be used wherever a Beverage
is expected.
Decorating for New Behavior

ConcreteDecorator classes inherit from the decorator base class, adding their unique behavior:
// Java Example
public class Milk extends BeverageDecorator {
public Milk(Beverage beverage) {
super(beverage);
}
@Override
public String getDescription() {
return beverage.getDescription() + ", Milk";
}
@Override
public double cost() {
return beverage.cost() + 0.30;
}
}
Here, Milk
adds milk to the beverage's description and cost. Inheritance ensures that each decorator has access to the original beverage's methods while modifying or extending their functionality.
Why Not Use Pure Inheritance?

The decorator pattern uses a combination of composition and inheritance because:
- Flexibility: Decorators allow for dynamic modifications at runtime, which inheritance doesn't support.
- Avoiding Class Explosion: Pure inheritance would lead to a combinatorial explosion of subclasses for each possible combination.
- Open-Closed Principle: Decorators make it possible to add behavior without changing existing classes.
💡 Note: Decorators provide a more granular approach to adding responsibilities, while inheritance creates a more rigid, often deeply nested class hierarchy.
Code Example: Decorator in Action

Here's a simple example of how you might implement decorators for a coffee shop system:
// Java Example
public interface Beverage {
String getDescription();
double cost();
}
public class Espresso implements Beverage {
@Override
public String getDescription() {
return "Espresso";
}
@Override
public double cost() {
return 1.99;
}
}
public abstract class BeverageDecorator implements Beverage {
protected Beverage beverage;
public BeverageDecorator(Beverage beverage) {
this.beverage = beverage;
}
@Override
public String getDescription() {
return beverage.getDescription();
}
@Override
public double cost() {
return beverage.cost();
}
}
public class Milk extends BeverageDecorator {
public Milk(Beverage beverage) {
super(beverage);
}
@Override
public String getDescription() {
return beverage.getDescription() + ", Milk";
}
@Override
public double cost() {
return beverage.cost() + 0.30;
}
}
public class Syrup extends BeverageDecorator {
public Syrup(Beverage beverage) {
super(beverage);
}
@Override
public String getDescription() {
return beverage.getDescription() + ", Syrup";
}
@Override
public double cost() {
return beverage.cost() + 0.50;
}
}
public class CoffeeShop {
public static void main(String[] args) {
Beverage beverage = new Espresso();
System.out.println(beverage.getDescription() + " $" + beverage.cost());
beverage = new Milk(beverage);
System.out.println(beverage.getDescription() + " $" + beverage.cost());
beverage = new Syrup(beverage);
System.out.println(beverage.getDescription() + " $" + beverage.cost());
}
}
This example demonstrates how decorators can wrap around an object, adding new behaviors or modifying existing ones at runtime.
💡 Note: This approach allows for a clean, organized, and extensible way to add features to objects, mimicking inheritance while remaining more flexible.
Recapping the essence of decorators:
- They provide a solution for adding behavior to an object without affecting other objects from the same class.
- Incorporating inheritance, decorators ensure type consistency, allowing for a decorator to behave just like the decorated object.
- By extending decorators with inheritance, developers can maintain a clean, single-focus design while still achieving dynamic extension.
By understanding and utilizing decorator classes, developers can build software systems that are not only flexible but also maintainable and scalable, adhering to the principles of good object-oriented design.
Can I combine multiple decorators on a single object?

+
Yes, one of the benefits of the decorator pattern is the ability to stack multiple decorators, allowing for a highly customizable object.
Is the decorator pattern better than inheritance?

+
It depends. Decorators offer flexibility and reduced class coupling, but for some cases where a static subclassing relationship is appropriate, inheritance might be the better choice.
How do decorators affect object creation?

+
Decorators can create a complex object structure at runtime, potentially leading to more instances in memory. However, they often lead to cleaner, more readable code.